2024. 7. 1. 20:26ㆍFlutter/Flutter Programming
플러터(Flutter) 애플리케이션에서 날짜와 시간을 다루는 것은 매우 중요한 부분입니다. 이번 글에서는 플러터에서 DateTime을 다루는 기본적인 방법과 문자열로부터 DateTime 객체를 파싱하는 방법에 대해 알아보겠습니다.
1. DateTime 클래스 소개
DateTime 클래스는 Dart 언어에서 제공하는 기본적인 클래스로, 날짜와 시간을 표현하고 다룰 수 있게 해줍니다. 플러터 애플리케이션에서 날짜와 시간을 효율적으로 관리하기 위해 이 클래스를 활용합니다. DateTime 클래스의 주요 기능은 다음과 같습니다:
- 날짜와 시간의 생성 및 표현
- 날짜와 시간의 비교
- 날짜와 시간 간의 연산 (더하기, 빼기 등)
- 날짜와 시간의 포맷 변경
2. DateTime 객체 생성 및 기본 사용법
DateTime 클래스를 사용하여 특정 날짜와 시간을 생성하고 현재 시간을 가져오는 방법을 살펴봅시다.
현재 날짜와 시간 가져오기
import 'package:flutter/material.dart';
void main() {
DateTime now = DateTime.now();
print('현재 날짜와 시간: $now');
}
위 예제에서 DateTime.now()를 사용하여 현재 시간을 가져와서 출력합니다.
특정 날짜와 시간 설정하기
import 'package:flutter/material.dart';
void main() {
DateTime customDateTime = DateTime(2023, 7, 1, 14, 30);
print('특정 날짜와 시간: $customDateTime');
}
위 예제에서는 DateTime(year, month, day, hour, minute) 생성자를 사용하여 특정 날짜와 시간을 설정합니다.
3. DateTime 포맷 변경
DateTime 객체를 원하는 포맷의 문자열로 변경하는 방법을 살펴봅시다. 이를 위해 intl 패키지를 사용할 수 있습니다.
intl 패키지 추가하기
먼저, pubspec.yaml 파일에 intl 패키지를 추가합니다.
dependencies:
flutter:
sdk: flutter
intl: ^0.17.0
DateTime 포맷 변경 예제
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() {
DateTime now = DateTime.now();
String formattedDate = DateFormat('yyyy-MM-dd').format(now);
print('포맷 변경: $formattedDate');
}
위 예제에서는 DateFormat('yyyy-MM-dd')를 사용하여 DateTime 객체를 'yyyy-MM-dd' 포맷의 문자열로 변경합니다.
4. DateTime 파싱하기
문자열로부터 DateTime 객체를 생성하려면 DateTime.parse() 메서드를 사용합니다.
DateTime 파싱 예제
import 'package:flutter/material.dart';
void main() {
String dateString = '2023-07-01 14:30:00';
DateTime parsedDateTime = DateTime.parse(dateString);
print('파싱 결과: $parsedDateTime');
}
위 예제에서는 DateTime.parse()를 사용하여 문자열 '2023-07-01 14:30:00'을 DateTime 객체로 파싱합니다.
5. 종합 예제: DatePicker 사용하기
마지막으로, DateTime을 사용하여 DatePicker를 구현하는 예제를 살펴봅시다.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
DateTime selectedDate = DateTime.now();
Future<void> _selectDate(BuildContext context) async {
final DateTime picked = await showDatePicker(
context: context,
initialDate: selectedDate,
firstDate: DateTime(2020),
lastDate: DateTime(2025),
);
if (picked != null && picked != selectedDate) {
selectedDate = picked;
print('선택한 날짜: $selectedDate');
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('DatePicker 예제')),
body: Center(
child: ElevatedButton(
onPressed: () => _selectDate(context),
child: Text('날짜 선택'),
),
),
),
);
}
}
위 예제에서는 showDatePicker() 함수를 사용하여 사용자가 날짜를 선택할 수 있는 DatePicker를 구현합니다.
결론
플러터에서 DateTime을 다루는 기본적인 방법과 문자열로부터 DateTime 객체를 파싱하는 방법에 대해 알아보았습니다.
이를 통해 애플리케이션에서 날짜와 시간을 효과적으로 관리하고 사용할 수 있을 것입니다.
추가적으로 필요한 기능이나 활용 방법에 따라 DateTime 클래스를 활용하여 다양한 날짜 및 시간 기능을 구현할 수 있습니다.
수발가족을 위한 일기장 “나비일기장”
https://play.google.com/store/apps/details?id=com.maccrey.navi_diary_release
구글플레이 앱 배포의 시작! 비공개테스트 20명의 테스터모집을 위한 앱 "테스터 쉐어"
https://play.google.com/store/apps/details?id=com.maccrey.tester_share_release
카카오톡 오픈 채팅방
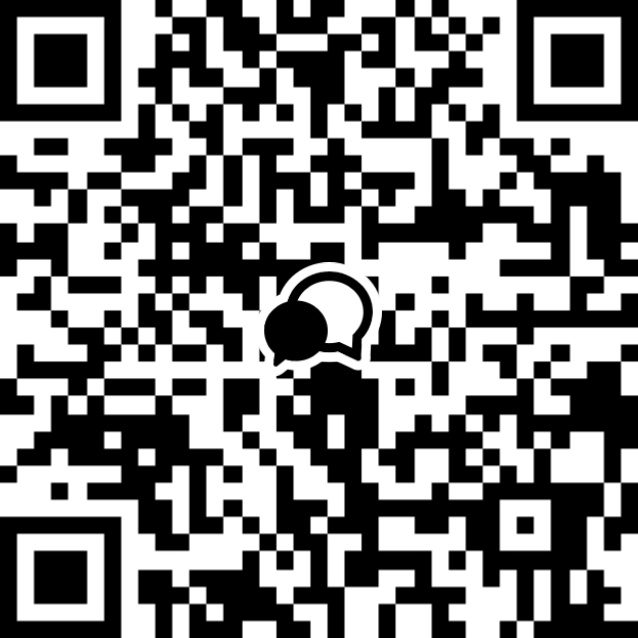
'Flutter > Flutter Programming' 카테고리의 다른 글
플러터에서 Get It 패키지 사용하기: 심층 가이드 (0) | 2024.07.02 |
---|---|
플러터에서 freezed 패키지 사용하기: 심층 가이드 (0) | 2024.07.02 |
플러터에서 SlidingUpPanel 패키지 사용 방법과 옵션 정리 (0) | 2024.07.01 |
플러터에서 애니메이션 카운트다운 구현하기: slide_countdown 패키지 사용법 가이드 (2) | 2024.07.01 |
플러터에서 간편하게 데이터 저장하기: shared_preferences 패키지 사용법 가이드 (0) | 2024.07.01 |