Flutter에서 http 패키지는 RESTful API와 통신하는 데 주로 사용됩니다.
이 블로그에서는 http 패키지의 기본 사용법부터 다양한 옵션과 고급 기능에 대해 알아보겠습니다.
1. HTTP 패키지 소개
http 패키지는 Flutter 애플리케이션이 웹 서버와 통신할 수 있게 해주는 간단하고 직관적인 API를 제공합니다. 이 패키지를 사용하면 HTTP 요청을 쉽게 만들고, 응답 데이터를 처리할 수 있습니다.
2. HTTP 패키지 설치
먼저, pubspec.yaml 파일에 http 패키지를 추가합니다.
dependencies:
flutter:
sdk: flutter
http: ^0.13.4
그런 다음, 패키지를 설치합니다.
flutter pub get
3. 기본 사용법
GET 요청
GET 요청은 서버로부터 데이터를 가져올 때 사용됩니다.
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('HTTP GET Example')),
body: Center(
child: FutureBuilder(
future: fetchData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
}
return Text('Data: ${snapshot.data}');
} else {
return CircularProgressIndicator();
}
},
),
),
),
);
}
Future<String> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
return json.decode(response.body)['title'];
} else {
throw Exception('Failed to load data');
}
}
}
POST 요청
POST 요청은 서버에 데이터를 보낼 때 사용됩니다.
Future<void> postData() async {
final response = await http.post(
Uri.parse('https://jsonplaceholder.typicode.com/posts'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'foo',
'body': 'bar',
'userId': '1',
}),
);
if (response.statusCode == 201) {
print('Success: ${response.body}');
} else {
throw Exception('Failed to post data');
}
}
PUT 요청
PUT 요청은 서버에 데이터를 업데이트할 때 사용됩니다.
Future<void> updateData() async {
final response = await http.put(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'id': '1',
'title': 'foo',
'body': 'bar',
'userId': '1',
}),
);
if (response.statusCode == 200) {
print('Success: ${response.body}');
} else {
throw Exception('Failed to update data');
}
}
DELETE 요청
DELETE 요청은 서버에서 데이터를 삭제할 때 사용됩니다.
Future<void> deleteData() async {
final response = await http.delete(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
);
if (response.statusCode == 200) {
print('Success: ${response.body}');
} else {
throw Exception('Failed to delete data');
}
}
4. HTTP 요청 옵션
헤더 설정
헤더를 사용하여 요청에 추가 정보를 포함할 수 있습니다.
final response = await http.get(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Authorization': 'Bearer your_api_token',
},
);
쿼리 파라미터
쿼리 파라미터를 사용하여 요청 URL에 추가 매개변수를 포함할 수 있습니다.
final response = await http.get(
Uri.parse('https://jsonplaceholder.typicode.com/posts?userId=1'),
);
바디 데이터
POST, PUT 요청 등에서 바디 데이터를 JSON 형식으로 포함할 수 있습니다.
final response = await http.post(
Uri.parse('https://jsonplaceholder.typicode.com/posts'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'foo',
'body': 'bar',
'userId': '1',
}),
);
5. 고급 기능
에러 핸들링
HTTP 요청에서 발생할 수 있는 다양한 오류를 처리해야 합니다.
Future<String> fetchData() async {
try {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
return json.decode(response.body)['title'];
} else {
throw Exception('Failed to load data');
}
} catch (e) {
print('Error: $e');
return 'Error occurred';
}
}
타임아웃 설정
HTTP 요청에 타임아웃을 설정할 수 있습니다.
final response = await http.get(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
).timeout(Duration(seconds: 10));
인증
Bearer 토큰과 같은 인증 정보를 헤더에 포함할 수 있습니다.
final response = await http.get(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Authorization': 'Bearer your_api_token',
},
);
6. HTTP 사용 시 주의사항
- 보안: 민감한 데이터를 HTTP 요청에 포함할 때는 반드시 HTTPS를 사용하세요.
- 에러 처리: 모든 HTTP 요청에 대해 적절한 에러 처리를 구현하세요.
- 비동기 처리: HTTP 요청은 비동기적으로 처리되므로, async와 await를 적절히 사용하여 코드를 작성하세요.
7. 결론
http 패키지는 Flutter에서 RESTful API와 통신하는 데 매우 유용한 도구입니다.
이 블로그에서는 기본 사용법부터 고급 기능까지 다루었습니다.
http 패키지를 사용하여 Flutter 애플리케이션에서 다양한 네트워크 작업을 효과적으로 수행해보세요!
https://play.google.com/store/apps/details?id=com.maccrey.navi_diary_release
구글플레이 앱 배포의 시작! 비공개테스트 20명의 테스터모집을 위한 앱 "테스터 쉐어"
https://play.google.com/store/apps/details?id=com.maccrey.tester_share_release
Tester Share [테스터쉐어] - Google Play 앱
Tester Share로 Google Play 앱 등록을 단순화하세요.
play.google.com
카카오톡 오픈 채팅방
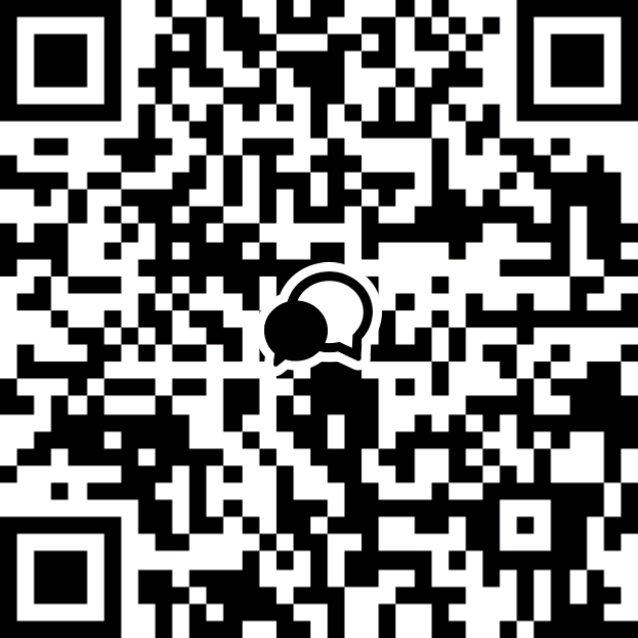
https://open.kakao.com/o/gsS8Jbzg
'Flutter > Flutter Programming' 카테고리의 다른 글
플러터 변수 범위: 로컬 변수 vs 파라미터 (심층 비교) (0) | 2024.06.19 |
---|---|
플러터에서 변수의 종류 (0) | 2024.06.19 |
플러터에서 Dialog 사용법과 옵션 (0) | 2024.06.19 |
플러터에서 Provider 패키지 사용법과 옵션[상태관리] (0) | 2024.06.19 |
플러터에서 easy_navigation 패키지 사용법 및 옵션 (0) | 2024.06.19 |